This is my loose collection of snippets, applications and commands, I use from day to day.
dot
dot is a graph description language. Assuming a image viewer like feh is installed
echo 'digraph { a -> b; b -> c; a -> c; }' | dot -Tpng | feh -
will show
snippet
remove empty lines from output
sed '/^\s*$/d'
loc
count lines of code (simple)
find . -name '*.extension' | xargs wc -l
same as above without emtpy lines and comments for erlang files
$ find . -name '*.erl' | \
> xargs cat | \
> sed -e '/^%/d' -e '/^\s*$/d' | \
> wc -l
for elixir
projects
$ find . -type f \( -iname \*.ex -o -iname \*.eex -o -iname \*.exs \) | \
> grep -v deps | \
> xargs cat | \
> sed -e '/^\s*$/d' | \
> wc -l
bash
HISTSIZE=""
or
HISTSIZE="INFINITE"
will let the ~/.bash_history
grow infinitely. source
gentoo specific aliases
alias eqf='equery f'
alias equ='equery u'
alias eqh='equery h'
alias eqa='equery a'
alias eqb='equery b'
alias eql='equery l'
alias eqd='equery d'
alias eqg='equery g'
alias eqc='equery c'
alias eqk='equery k'
alias eqm='equery m'
alias eqy='equery y'
alias eqs='equery s'
alias eqw='equery w'
alias current="sudo genlop -c"
silver searcher
When you use the silver searcher code search, you can add a .ignore
file to the root folder of your application. This file is similar to .gitignore
files. Due to the double asterix is not working yet, a quick find can help you to find the files, which should be excluded. e.g.
$ find priv/static/libs/ -type f \( -name "*.js" -o -name "*.css" -o -name "*.map" \)
will find all files with the extension *.js
, *.css
, or *.map
. This is helpfull, when you want to exclude build artefacts.
git
pull all repositories located in sub directories, assuming there are only subdirectories in the current directory
reset a single file
git checkout HEAD -- fileName.xxx
aliases
common aliases
git config --global alias.co checkout
git config --global alias.br branch
git config --global alias.ci commit
git config --global alias.st status
reset a single file to head
git config --global alias.unstage "reset HEAD --"
git unstage file.txt
show graph
git config --global alias.l "log --graph --oneline --all"
cat diff to default browser
With diff2html a git diff
output can be transformed into html.
npm install -g diff2html
bcat is a tool for piping data to the standard browser.
git diff branch_1..branch_2 | diff2html -i stdin -o stdout | bcat
With an alias
alias bdiff="diff2html -i stdin -o stdout | bcat"
the call can be simplified to.
git diff branch_1..branch_2 | bdiff
If you want to see the history of a file, you can use
git log -p filename | bdiff
cat the content of a libreoffice or docx document to console
alias lcat="libreoffice --cat"
with
lcat document.docx | vim -
you can browse the text content in your favourite editor ;)
firefox addons
- VimFx - using vim commands for browsing
- MarkdownViewer - render markdown files
tmux
basic
tmux a
- atach<ctrl> b d
- detatch<shift>
- select text with left mouse bottom link<shift>
- paste text with right mouse bottom
Window
<ctrl> b c
- create new window<ctrl> b n
- next window
Pane
<ctrl> b x
- kill pane<ctrl> b "
- create horizontal pane<ctrl> b %
- create vertical pane
batch
for /F %L in (commandParameters.txt) do echo %L
- Execute command for every line in given textfile (in this case theecho
command) / for command
vim
- vimsheed - a good summary of vim commands.
movement
basic movement
k
- upj
- downh
- leftl
- right
jumps
0
- beginning of a line$
- end of a linew
- beginning of a world)
- forward one sentence(
- backward one sentence
visual
vi'
- select inner text between two'
<C-V>
- select columnI
- insert text -><ESC>
-> insert text in front of the selection
repeats
g
- global:g/Test/d
- delete all lines containingTest
:g!/Test/d
- delete all lines not containingTest
substitute
:%s/foo/bar/g
- replace allfoo
withbar
settings
:set ignorecase
- case insensitive search:set wrap
/:set nowrap
- enables / disables automatic line breaks
misc
u
- undo<ctrl> r
- redo:w !sudo tee %
- save file, if you forgotsudo
:so $MYVIMRC
- reload the current.vimrc
e $MYVIMRC
- edit the current.vimrc
haskell
Preconditions
- ghci / cabal / stack installed
stack new projectName simple
- start a new simple haskell project
after changed into project folder
stack setup
- init projectstack build
- build the projectstack exec projectName
- start programmstack ghci
- load project aware ghci
ghci
:set -XOverloadedStrings
- activate overloaded string extension
erlang / elixir
observer
Erlang
has to be emerged with smp
and wxwidgets
use flag. For elixir
you can start the observer
with
$ iex
Erlang/OTP 20 [erts-9.1] [source] [64-bit] [smp:8:8] [async-threads:10]
Interactive Elixir (1.5.2) - press Ctrl+C to exit (type h() ENTER for help)
iex(1)> :observer.start
:ok
iex(2)>
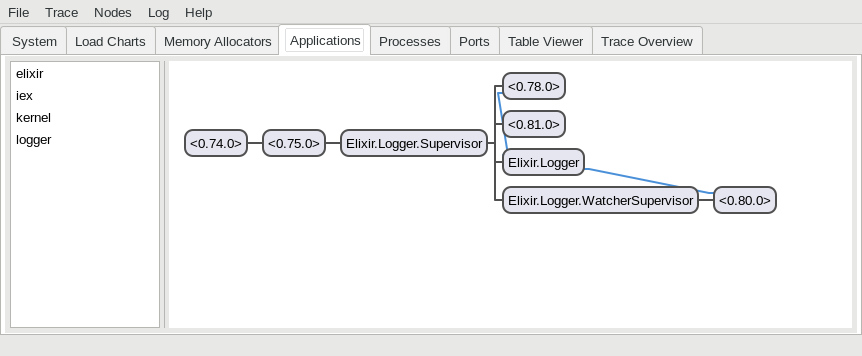
Windows batch programming
START "" http://localhost
- starts a default browser withhttp://localhost
or opens a new tab.
.NET / C#
Force to copy an unused assembly
When the compiler sees no usage of a referenced assembly, it won’t be copied to the output folder. If you need for some reason the assembly in your output folder, you can create a dummy usage, which forces the compiler to copy the assembly.
private static void Dummy()
{
Action<Type> doesNothingWith = _ => { };
var anyType = typeof(ReferencedAssembly.SomeClass);
doesNothingWith(anyType);
}
Development stack
Sometimes it is usefull, to start a build from the command line. A make_debugBuild.bat
can look like
call "C:\Program Files (x86)\Microsoft Visual Studio 14.0\Common7\Tools\VsDevCmd.bat"
msbuild SolutionName.sln /p:Configuration=Debug
For a make_releaseBuild.bat
you can just change the msbuild Configuration
parameter.
call "C:\Program Files (x86)\Microsoft Visual Studio 14.0\Common7\Tools\VsDevCmd.bat"
msbuild SolutionName.sln /p:Configuration=Release
To delete common generated / build folders from command line, you can use a cleanup.bat
like
FOR /F "tokens=*" %%G IN ('DIR /B /AD /S obj') DO RMDIR /S /Q "%%G"
FOR /F "tokens=*" %%G IN ('DIR /B /AD /S bin') DO RMDIR /S /Q "%%G"
REM RMDIR /S /Q AdditionalFolderToDelete
Windows
Here comes the Windows related stuff
Chocolatey
A package manager for Windows.
Installation
Before the installation you must allow remote scripts to run. Start a powershell with administrator rights
Set-ExecutionPolicy RemoteSigned
iwr https://chocolatey.org/install.ps1 -UseBasicParsing | iex
Ack
choco install ack
misc
whatching amazon prime video from chromium on a gentoo system via chromecast
At first the widevine
use flag for chromium
has to be enabled. It enables the closed source capability of chromium, used by Amazon Prime Video and Netflix. The chrome://flags/#load-media-router-component-extension
has to be enabled
. With this configuration you can go to prime videos and cast the tab while playing.
In the long run a fire tv stick is a better fit, because it comes from the same house.
setup system date
# date 041513122017
Sat Apr 15 13:12:00 CEST 2017
xclip
cat id_rsa.pub | xclip -selection clipboard
brightness
$ sudo echo 800 > /sys/class/backlight/intel_backlight/brightness
sets the current brightness to 800
. (1500 is default)
gcalcli
Working with the Google calendar on console
put a .gcalclirc
in users home directory
--client_id=<client_id>
--client_secret=<client-secret>
--locale=de_DE.utf8
--defaultCalendar=<calendar name>
Set up some convenient aliases
alias week="gcalcli calw"
alias month="gcalcli calm"
alias todo="gcalcli agenda"
Get the next three weeks
week 3
typescript
Automatic compile typescript application.
First create a make target like
.PHONY: tsc
tsc:
rm *build_artefacts* || true
tsc --build tsconfig.json
Then create an alias
like
alias notify='while true; do inotifywait -re modify *root_of_ts_sources' && make tsc; done'
which deletes the build artefacts if needed, and recompile the application.